English
106. Construct Binary Tree from Inorder and Postorder Traversal
Problem Statement
Given two integer arrays inorder
and postorder
where inorder
is the inorder traversal of a binary tree and postorder
is the postorder traversal of the same tree, construct and return the binary tree.
Example 1:
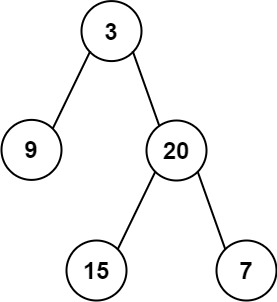
Input: inorder = [9,3,15,20,7], postorder = [9,15,7,20,3]
Output: [3,9,20,null,null,15,7]
Example 2:
Input: inorder = [-1], postorder = [-1]
Output: [-1]
Constraints:
1 <= inorder.length <= 3000
postorder.length == inorder.length
-3000 <= inorder[i], postorder[i] <= 3000
inorder
andpostorder
consist of unique values.- Each value of
postorder
also appears ininorder
. inorder
is guaranteed to be the inorder traversal of the tree.postorder
is guaranteed to be the postorder traversal of the tree.
Solution:
go
package main
// Definition for a binary tree node.
type TreeNode struct {
Val int
Left *TreeNode
Right *TreeNode
}
func buildTree(inorder []int, postorder []int) *TreeNode {
mapInOrder := make(map[int]int)
for i, v := range inorder {
mapInOrder[v] = i
}
var helper func(int, int) *TreeNode
helper = func(left, right int) *TreeNode {
if left > right {
return nil
}
pop := postorder[len(postorder)-1]
postorder = postorder[:len(postorder)-1]
root := &TreeNode{Val: pop}
mid := mapInOrder[pop]
root.Right = helper(mid+1, right)
root.Left = helper(left, mid-1)
return root
}
return helper(0, len(inorder)-1)
}
...