English
234. Palindrome Linked List
Problem Statement
Given the head
of a singly linked list, return true
if it is a palindrome or false
otherwise.
Example 1:

Input: head = [1,2,2,1]
Output: true
Example 2:
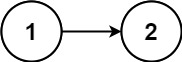
Input: head = [1,2]
Output: false
Constraints:
- The number of nodes in the list is in the range
[1, 105]
. 0 <= Node.val <= 9
Follow up: Could you do it in
O(n)
time and O(1)
space? Solution:
go
package main
// Definition for singly-linked list.
type ListNode struct {
Val int
Next *ListNode
}
func isPalindrome(head *ListNode) bool {
if head == nil {
return true
}
// find the middle node
slow, fast := head, head
for fast.Next != nil && fast.Next.Next != nil {
slow = slow.Next
fast = fast.Next.Next
}
// reverse the second half
var prev *ListNode
curr := slow.Next
for curr != nil {
next := curr.Next
curr.Next = prev
prev = curr
curr = next
}
// compare the first half and the reversed second half
for prev != nil {
if head.Val != prev.Val {
return false
}
head = head.Next
prev = prev.Next
}
return true
}
...