English
141. Linked List Cycle
Problem Statement
Given head
, the head of a linked list, determine if the linked list has a cycle in it.
There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following theĀ next
Ā pointer. Internally, pos
Ā is used to denote the index of the node thatĀ tail'sĀ next
Ā pointer is connected to.Ā Note thatĀ pos
Ā is not passed as a parameter.
ReturnĀ true
if there is a cycle in the linked list. Otherwise, return false
.
Ā
Example 1:
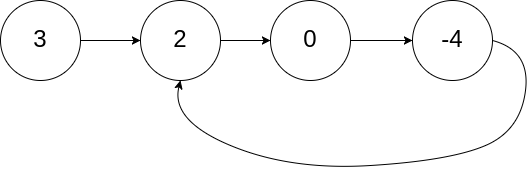
Input: head = [3,2,0,-4], pos = 1
Output: true
Explanation: There is a cycle in the linked list, where the tail connects to the 1st node (0-indexed).
Example 2:
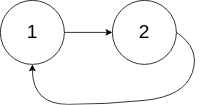
Input: head = [1,2], pos = 0
Output: true
Explanation: There is a cycle in the linked list, where the tail connects to the 0th node.
Example 3:

Input: head = [1], pos = -1
Output: false
Explanation: There is no cycle in the linked list.
Ā
Constraints:
- The number of the nodes in the list is in the range
[0, 104]
. -105 <= Node.val <= 105
pos
is-1
or a valid index in the linked-list.
Ā
Follow up: Can you solve it using O(1)
(i.e. constant) memory?
Solution:
go
package main
// Definition for singly-linked list.
type ListNode struct {
Val int
Next *ListNode
}
func hasCycle(head *ListNode) bool {
if head == nil {
return false
}
slow, fast := head, head
for fast != nil && fast.Next != nil {
slow = slow.Next
fast = fast.Next.Next
if slow == fast {
return true
}
}
return false
}
...