English
203. Remove Linked List Elements
Problem Statement
Given the head
of a linked list and an integer val
, remove all the nodes of the linked list that has Node.val == val
, and return the new head.
Example 1:
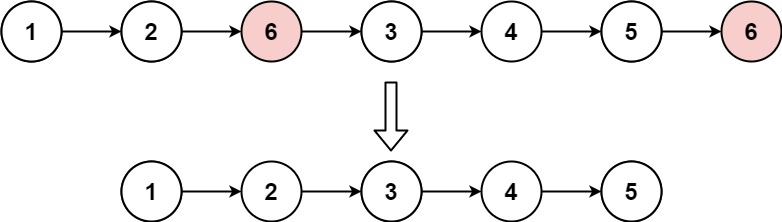
Input: head = [1,2,6,3,4,5,6], val = 6
Output: [1,2,3,4,5]
Example 2:
Input: head = [], val = 1
Output: []
Example 3:
Input: head = [7,7,7,7], val = 7
Output: []
Constraints:
- The number of nodes in the list is in the range
[0, 104]
. 1 <= Node.val <= 50
0 <= val <= 50
Solution:
go
package main
// Definition for singly-linked list.
type ListNode struct {
Val int
Next *ListNode
}
// Iterative
func removeElements(head *ListNode, val int) *ListNode {
if head == nil {
return nil
}
for head != nil && head.Val == val {
head = head.Next
}
if head == nil {
return nil
}
for p := head; p.Next != nil; {
if p.Next.Val == val {
p.Next = p.Next.Next
} else {
p = p.Next
}
}
return head
}
// Recursive
func removeElementsRecursive(head *ListNode, val int) *ListNode {
if head == nil {
return nil
}
head.Next = removeElementsRecursive(head.Next, val)
if head.Val == val {
return head.Next
}
return head
}
...